In Android development, One of the famous open source library is Butterknife. What is ButterKnife? A Library that binds your Android views which uses annotation process. built by Jake Wharton.
- Very light weight.
- Eliminates long codes in finding your Android views.
- It uses annotation such as @Bind, @OnClick and more.
- Clean code and organize.
- Binds String resources.
Personally I always includes this library in most of my projects , I highly recommend you guys to use this, it will improve your speed in production.
you can add this library using Gradle system of Android studio
dependencies { compile 'com.jakewharton:butterknife:7.0.1' }
After syncing your Gradle, you can now use Butterknife in your project.
Here's My Example Android App that can get the Sum or Diff of two numbers.
1.MainActivity.java
package com.example.uge.butterknifesample; import android.support.v7.app.AppCompatActivity; import android.os.Bundle;; import android.view.inputmethod.InputMethodManager; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; import butterknife.Bind; import butterknife.ButterKnife; import butterknife.OnClick; public class MainActivity extends AppCompatActivity { @Bind(R.id.firstNum) EditText firstNum; @Bind(R.id.secondNum) EditText secondNum; @Bind(R.id.answerText) TextView answerTxt; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); ButterKnife.bind(this); } @OnClick(R.id.sumBtn) void getTheSumOfTwoNumbers(){ if(validateEditText()){ int fValue = Integer.parseInt(firstNum.getText().toString()); int sValue = Integer.parseInt(secondNum.getText().toString()); int sumValue = fValue + sValue; answerTxt.setText("Sum: " + sumValue); }else{ Toast.makeText(this, "Please Input the Values in the EditText(s)", Toast.LENGTH_SHORT).show(); } hideKeyboard(); } @OnClick(R.id.diffBtn) void getTheDiffOfTwoNumbers(){ if(validateEditText()){ int fValue = Integer.parseInt(firstNum.getText().toString()); int sValue = Integer.parseInt(secondNum.getText().toString()); int diffValue = fValue - sValue; answerTxt.setText("Diff: " + diffValue); }else{ Toast.makeText(this, "Please Input the Values in the EditText(s)", Toast.LENGTH_SHORT).show(); } hideKeyboard(); } public Boolean validateEditText(){ //The Two EditText must have a value if(firstNum.getText().toString().length() > 0 && secondNum.getText().toString().length() > 0){ return true; }else{ return false; } } public void hideKeyboard(){ InputMethodManager imm = (InputMethodManager) getSystemService(INPUT_METHOD_SERVICE); if(imm.isAcceptingText()) { // verify if the soft keyboard is open imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0); } } }
2. Layout (activity_main.xml)
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_margin="15dp" tools:context="com.example.uge.butterknifesample.MainActivity"> <EditText android:id="@+id/firstNum" android:layout_width="match_parent" android:layout_height="45dp" android:inputType="number" android:hint="First Number" /> <EditText android:id="@+id/secondNum" android:layout_width="match_parent" android:layout_height="45dp" android:hint="Second Number" android:inputType="number" android:layout_below="@+id/firstNum" /> <Button android:id="@+id/sumBtn" android:layout_width="match_parent" android:layout_height="45dp" android:text="Sum" android:layout_below="@+id/secondNum" android:layout_margin="5dp"/> <TextView android:id="@+id/orText" android:layout_below="@+id/sumBtn" android:gravity="center" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="OR" android:layout_margin="5dp"/> <Button android:id="@+id/diffBtn" android:layout_width="match_parent" android:layout_height="45dp" android:text="Difference" android:layout_below="@+id/orText" android:layout_margin="5dp"/> <TextView android:id="@+id/answerText" android:textSize="20sp" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true"/> </RelativeLayout>
Here's the OUTPUT:
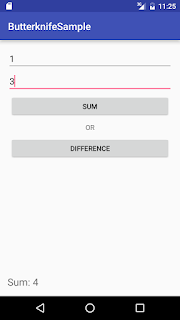
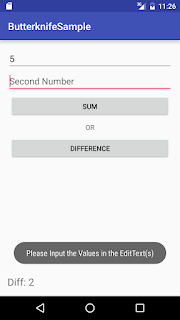
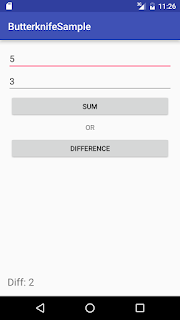
You can download the sources code here: ButterknifeSample
for automation of binding views in Butterknife you can use Butterknife zelezny