Ormlite is an open source software framework that provides lightweight object relational mapping (ORM) between Java classes and SQL databases. It supports JDBC databases as well as Android mobile platform according. To see more documentations about Ormlite visit this (http://ormlite.com/)
Okay lets Start the Project!! :D
1st add this to your dependencies
Download Jar here
dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) compile 'com.android.support:appcompat-v7:21.0.3' compile files('libs/ormlite-android-4.48.jar') compile files('libs/ormlite-core-4.48.jar') }
1.MainActivity.java
package com.example.user.ormlite; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; import com.j256.ormlite.android.apptools.OpenHelperManager; import com.j256.ormlite.dao.RuntimeExceptionDao; import java.util.List; public class MainActivity extends ActionBarActivity { DataHelper dbHelper; Button save; Button viewAll; Button delete; EditText student_name; EditText student_lastname; EditText student_age; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //Get All Widget save = (Button) findViewById(R.id.btn_save); viewAll = (Button) findViewById(R.id.btn_view); delete = (Button) findViewById(R.id.btn_delete); student_name = (EditText) findViewById(R.id.name); student_lastname = (EditText) findViewById(R.id.surname); student_age = (EditText) findViewById(R.id.age); innit(); } public void innit(){ //OpenHelper of DatabaseHelper dbHelper = (DataHelper) OpenHelperManager.getHelper(this,DataHelper.class); final RuntimeExceptionDao<student integer=""> studDao = dbHelper.getStudRuntimeExceptionDao(); save.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { if(student_name.getText().toString().length() > 0 && student_lastname.getText().toString().length() > 0 && student_age.getText().toString().length() > 0) { studDao.create(new Student(student_name.getText().toString(), student_lastname.getText().toString(), Integer.parseInt(student_age.getText().toString()))); student_name.setText(""); student_lastname.setText(""); student_age.setText(""); Toast.makeText(MainActivity.this, "Student Saved", Toast.LENGTH_SHORT).show(); } else Toast.makeText(MainActivity.this, "Please Complete all Inputs", Toast.LENGTH_SHORT).show(); } }); viewAll.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { String display = ""; List<student> students = studDao.queryForAll(); for(Student holder : students){ display += holder.getFname() + " " + holder.getLname() + " " +holder.getAge() + "\n"; } Toast.makeText(MainActivity.this,"Students:" + display, Toast.LENGTH_SHORT).show(); } }); delete.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { List<student> students = studDao.queryForAll(); studDao.delete(students); Toast.makeText(MainActivity.this,"Deleted", Toast.LENGTH_SHORT).show(); } }); } public void onDestroy(){ super.onDestroy(); OpenHelperManager.releaseHelper(); } }
2.Student.java Declaring your Database Table
import com.j256.ormlite.field.DatabaseField; import com.j256.ormlite.table.DatabaseTable; /** * Created by Eugene Alvizo on 1/4/2015. */ public class Student { //Database Field for Table Student @DatabaseField (generatedId = true) private int id; @DatabaseField private String fname; @DatabaseField private String lname; @DatabaseField private int age; //Constructors public Student() { //Adding a No-Argument-Constructor } public Student(String fname, String lname, int age) { this.fname = fname; this.lname = lname; this.age = age; } public Student(int id, String fname, String lname, int age) { this.id = id; this.fname = fname; this.lname = lname; this.age = age; } //Getters and Setters public int getId() { return id; } public void setId(int id) { this.id = id; } public String getFname() { return fname; } public void setFname(String fname) { this.fname = fname; } public String getLname() { return lname; } public void setLname(String lname) { this.lname = lname; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
package com.example.user.ormlite; import android.content.Context; import android.database.sqlite.SQLiteDatabase; import com.j256.ormlite.android.apptools.OrmLiteSqliteOpenHelper; import com.j256.ormlite.dao.Dao; import com.j256.ormlite.dao.RuntimeExceptionDao; import com.j256.ormlite.support.ConnectionSource; import com.j256.ormlite.table.TableUtils; import java.sql.SQLException; /** * Created by Eugene Alvizo on 1/4/2015. */ public class DataHelper extends OrmLiteSqliteOpenHelper { private Context context; private static final String DATABASE_NAME = "Student"; private static final int DATABASE_VERSION = 1; private RuntimeExceptionDao<student integer=""> studRuntimeDAO = null; public DataHelper(Context context) { super(context, DATABASE_NAME, null, DATABASE_VERSION); this.context = context; } @Override public void onCreate(SQLiteDatabase sqLiteDatabase, ConnectionSource connectionSource) { try { TableUtils.createTable(connectionSource, Student.class); } catch (SQLException e){ e.printStackTrace(); } } @Override public void onUpgrade(SQLiteDatabase sqLiteDatabase, ConnectionSource connectionSource, int i, int i2) { try { TableUtils.dropTable(connectionSource, Student.class, true); onCreate(sqLiteDatabase,connectionSource); } catch (SQLException e) { e.printStackTrace(); } } public RuntimeExceptionDao<student integer=""> getStudRuntimeExceptionDao(){ if(studRuntimeDAO == null){ studRuntimeDAO = getRuntimeExceptionDao(Student.class); } return studRuntimeDAO; } }
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity" android:orientation="vertical"> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:gravity="center"> <EditText android:layout_width="180dp" android:layout_height="45dp" android:hint="Name" android:id="@+id/name"/> <EditText android:layout_width="180dp" android:layout_height="45dp" android:hint="Lastname" android:id="@+id/surname"/> <EditText android:layout_width="180dp" android:layout_height="45dp" android:hint="Age" android:inputType="number" android:id="@+id/age"/> <Button android:layout_width="180dp" android:layout_height="45dp" android:text="Save" android:id="@+id/btn_save"/> <Button android:layout_width="180dp" android:layout_height="45dp" android:text="View All" android:id="@+id/btn_view"/> <Button android:layout_width="180dp" android:layout_height="45dp" android:text="Delete All" android:id="@+id/btn_delete"/> </LinearLayout> </LinearLayout>
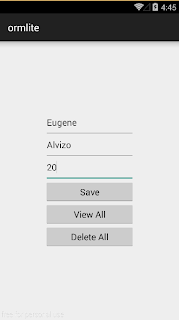
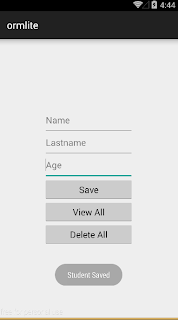
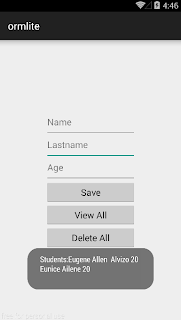
Download Source code (Android Studio Project) reference
Thanks for your sharing. It is so clear and successful expression.
ReplyDeleteThanks, I hope it helps in your current project :)
Deletevery clear but i have the error : incompatible types
ReplyDeleterequired : object found Student
for :
for(Student holder : students){
display += holder.getFname() + " " + holder.getLname() + " " +holder.getAge() + "\n";
}
you must have a class for the object in the for loop and it must the same objects with current holder.
DeleteHi! where is the route to you .sql?? i need to see it
ReplyDelete